해당 글은 김영한님의 <스프링 입문> 강좌를 기반으로 작성되었습니다.
Static Content 방식
Static Content 구현해보기
- 아래의 hello-static.html파일을 resources/static 디렉토리에 넣는다
<!DOCTYPE HTML>
<html>
<head>
<title>static content</title>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8" />
</head>
<!DOCTYPE HTML>
<body>
정적 컨텐츠입니다!
</body>
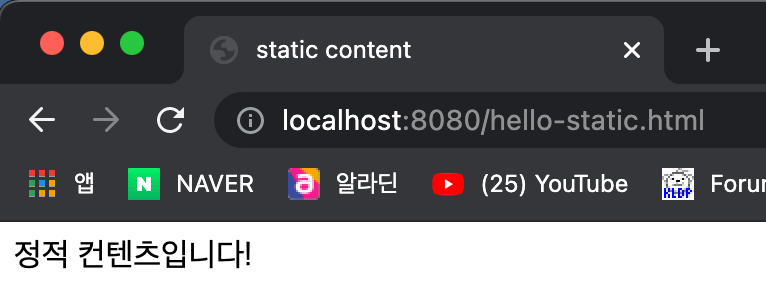
Static Content 구현 동작 이미지
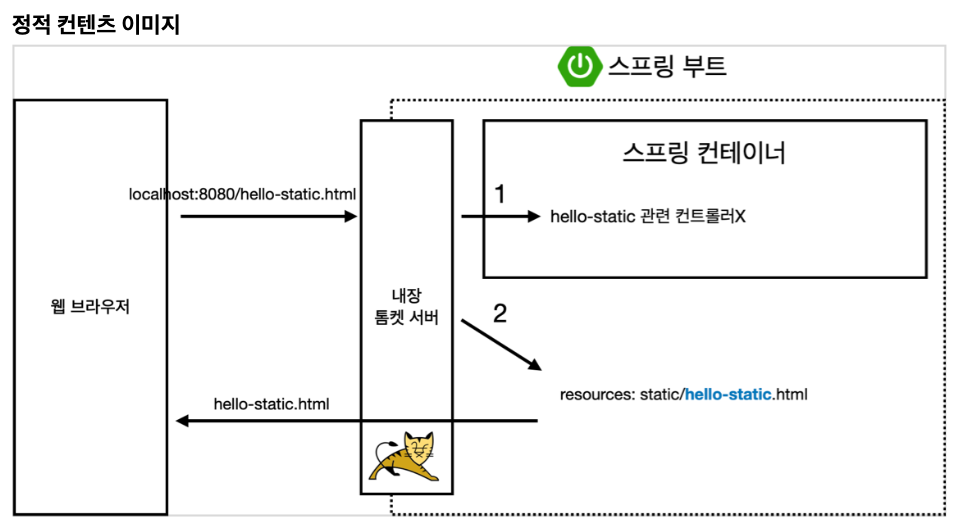
MVC(템플릿 엔진 사용) 방식
MVC
- Model,View,Controller의 약자로 각각의 역할을 나누어 본인의 일에만 집중하게 하는 방식이라고 한다. 코드를 보면 이해 할 수 있다.
Controller 구현
//Using MVC and Template Engine
@GetMapping("hello-mvc")
public String helloMvc(@RequestParam("name") String name, Model model){
model.addAttribute("name",name);
return "hello-template";
}
View 구현
- 위의 Controller에서 리턴해주는 “hello-template” 파일이 view역할을 한다.
- hello-template.html을 resources/templates에 생성한다.
<html xmlns:th="http://www.thymeleaf.org">
<body>
<p th:text="'hello ' + ${name}">hello! empty</p>
</body>
</html>
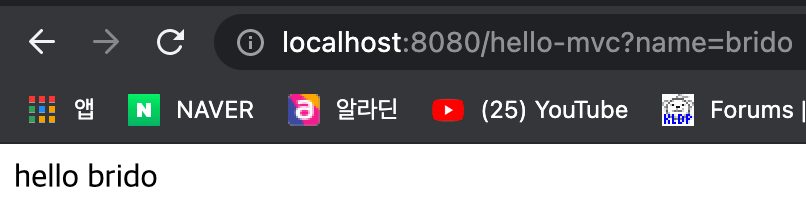
MVC 동작 그림
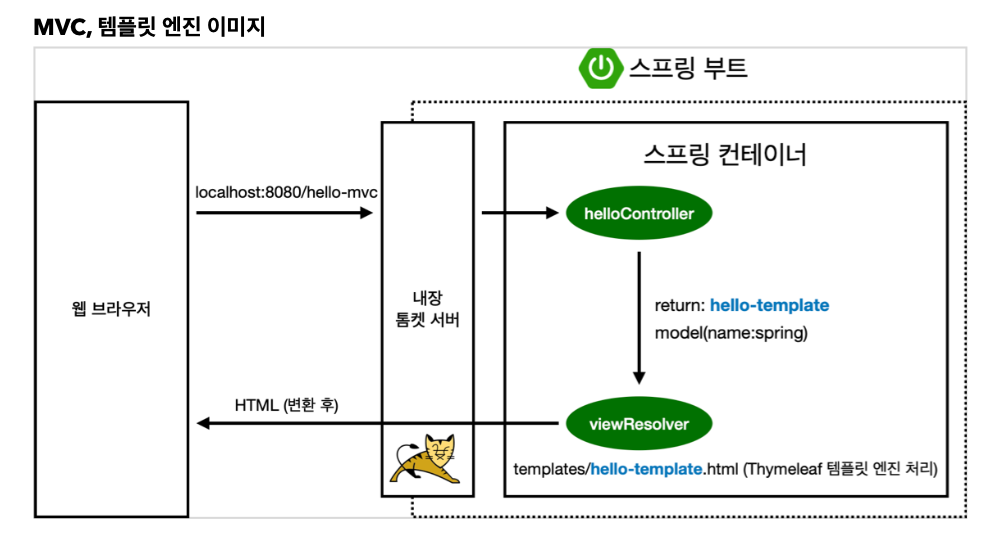
API 방식
@ResponseBody의 문자 반환
//Using API ver1 -> 메서드에서 String 반환
@GetMapping("hello-string")
@ResponseBody// 문자열 반환
public String helloString(@RequestParam("name") String name){
return "hello" + name;
}
- 해당 코드의 경우, hello + name의 값을 그대로 반환해주는 API 방식이며, 설명을 위해 가져온 예제라고 보면된다.
@ResponseBody의 객체 반환
//Using API ver2 - Using JSON -> 메서드에서 객체가 반환
@GetMapping("hello-api")
@ResponseBody // 객체 반환
public Hello helloApi(@RequestParam("name") String name){
Hello hello = new Hello();
hello.setName(name);
return hello;
}
static class Hello{
private String name;
//Getter and Setter -> java bean 표준
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
- 대부분의 경우 API를 사용하면 위의 방식을 사용한다.
- 위 방식은 서버와 서버간의 데이터 전송을 할때 JSON이라는 포맷에 객체를 전달하는 과정을 수행한다.
- 아래와 같이 url을 작성해 name에 brido라는 값을 전달하면, 해당 값을 전달받은 Hello객체가 JSON형태로 출력된다
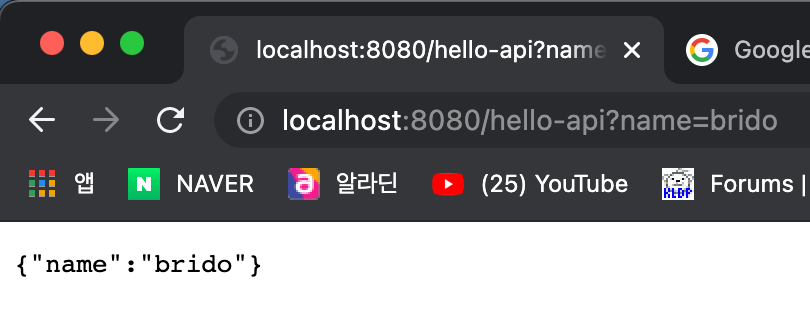
@ResponseBody 사용 원리
- HTTP Body에 데이터를 주입해서 전송한다.
- MVC 방식과 달리 view-resolver를 사용하지 않고 HttpMessageConverter가 동작한다.
- HttpMessageConverter는 다양한 Converter를 가지고 있으며 HTTP의 accept 헤더와 서버의 Controller 반환 타입을 보고 Converter를 결정한다.
- 문자열 처리는 StringHttpMessageConverter가 맡고 객체 처리는 스프링에선MappingJackson2HttpMessageConverter가 수행한다.